Exception Policies
The policies define how certain exception types are handled by the Exception Handling Application Block. A particular policy can have multiple exception types associated with it. Each exception type within the policy can then have one or more exception handlers. The exception handlers supplied with Enterprise Library include those for logging, wrapping, and replacing exceptions.
The ExceptionPolicy class
The ExceptionPolicy class serves as a simple service interface for the Exception Handling Application Block. It contains only one static, public method: HandleException. The method is responsible for calling the GetExceptionPolicy method which uses a Factory pattern implementation to create an instance of the ExceptionPolicyImpl class for the named policy that was passed in from the HandleException method.
An exception policy must be configured with a PostHandlingAction for every type of exception that it has been configured to handle. The value for the PostHandlingAction determines what the return value for the HandleException method will be. The possible values for the PostHandlingAction property are NotifyRethrow, None, and ThrowNewException. The default is NotifyRethrow.
Exception Handlers
An exception handler performs an action based on the existence of a specific exception type. These are the components that actually handle the exception. Four exception handlers are provided with Enterprise Library:
- LoggingExceptionHandler: This exception handler formats exception information, such as the message and the stack trace. Then the logging handler gives this information to the Enterprise Library Logging Application Block so that it can be published.
- ReplaceHandler: This exception handler replaces one exception with another.
- WrapperHandler: This exception handler wraps one exception around another.
- FaultContractExceptionHandler: This exception handler is designed for use at Windows Communication Foundation (WCF) service boundaries, and generates a new Fault Contract from the exception.
You can create your custom Exception Handler by implementing the IExceptionHanlder Interface.
Exception Message Formatters
ExceptionFormatters provides a flexible way to configure and format exception messages. Two implementations of the ExceptionFormatter class come with Enterprise Library: TextExceptionFormatter and XmlExceptionFormatter. You can also design you own custom ExceptionFormatter simply by creating a subclass of ExceptionFormatter and provide your own formatting implementation. Both derive from the abstract ExceptionFormatter base class
TextExceptionFormatter Class
The TextExceptionFormatter class allows the exception and the details about the exception to be written out as plain text to an instance of a TextWriter class. This can be useful for writing to log files
XmlExceptionFormatter Class
This works exactly like the TextExceptionFormatter except that it uses an XmlTextWriter to format the message as XML instead of strings of indented text.
Configuring and Using the Exception Handling Application Block
For using Exception handling application block in you applications you need to add reference reference to these three assemblies at a minimum:
• Microsoft.Practices.EnterpriseLibrary.Common.dll:
• Microsoft.Practices.EnterpriseLibrary.ExceptionHandling.dll:
• Microsoft.Practices.ObjectBuilder.dll:
If you intend on using the Logging Application Block to log exceptions as they occur, you will also need to add the following two assemblies to your project’s references:
• Microsoft.Practices.EnterpriseLibrary.Logging.dll:
• Microsoft.Practices.EnterpriseLibrary.ExceptionHandling.Logging.dll:
- Using the Enterprise library configuration console add a new Exception handling application block to the application.
- Create an exception policy by right clicking the Exception Handling Application Block and select New --> Exception Policy
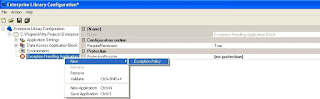
- Create a new exception type for this exception policy to handle, Right-click the MyPolicy node and select New --> Exception Type. Change the name to MyApplication Policy
Right Click the exception type and select New-->Exeption Type. Select Exception from the list.
- To add the logging handler to the exception type, Right-click the Exception node and select New --> Logging Handler.
- Click the Logging Hanlder under the Exception node and change the Category Source to General.
- Select the formatter type by clicking the eclipse button on the Formatter Type property of the Logging handler and select TextExceptionFormatter.
- Add the following code to throw an exception and handle that.
private void ThrowExceptionAndHandle()
{
try
{
throw new ArgumentException("Testing the Enterprise Library Application Block");
}
catch (Exception ex)
{
ExceptionPolicy.HandleException(ex, "MyApplication Policy");
}
} - Open the Application Event Veiwer to see the exception details.
1 comment:
Useful and concise article. Thank you!
Post a Comment